showAlert()
This page provides information about the showAlert()
function signature and parameters, which allows you to display a temporary toast-style alert message to the user for precisely 5 seconds. The duration of the alert message can't be modified.
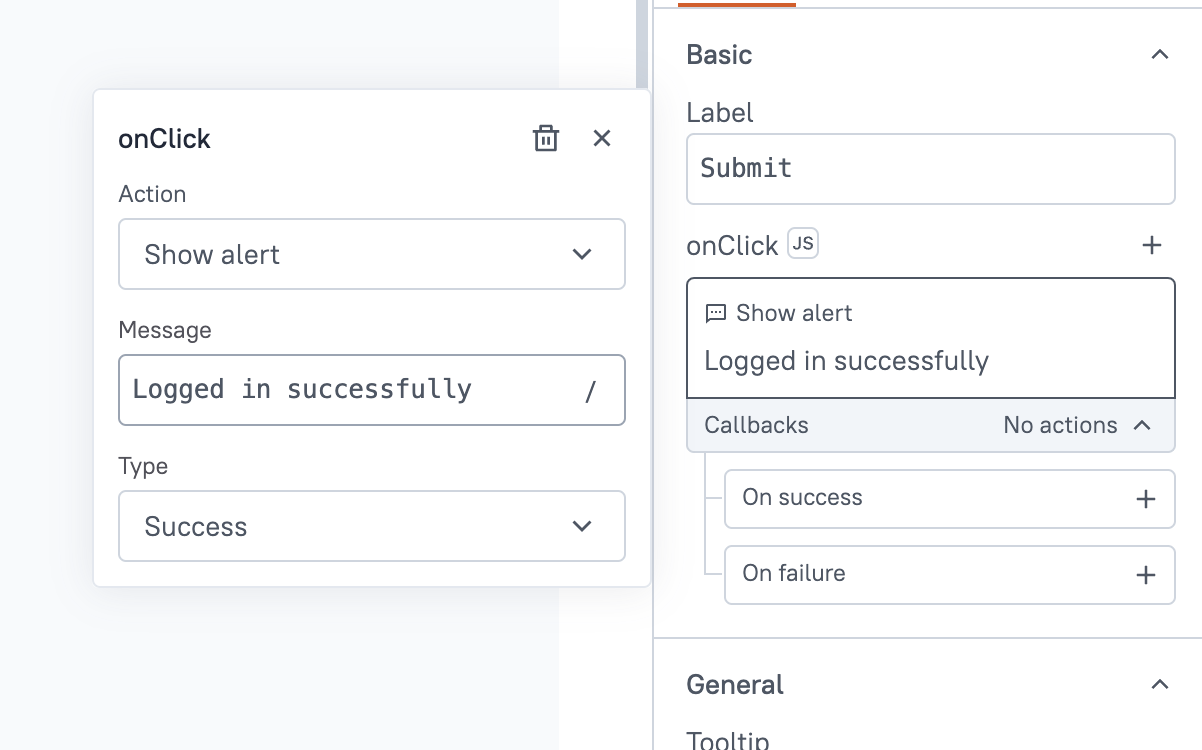
Signature
showAlert(message: string, type: string): Promise
Parameters
Below are the parameters required by the showAlert()
function to execute:
Message
The message
parameter is a string that contains the text displayed in the alert message. This message is shown to the user in a temporary toast alert. You can also pass dynamic data using JS.
Example:
{{showAlert('Welcome! ' + appsmith.user.username, 'info');}}
Type
The type
is an optional parameter that allows you to configure the type of the alert message, which determines the icon displayed in the toast message. It accepts the following string values:
info
success
error
warning
Example:
{{showAlert('Data submitted successfully', 'success');}}
Usage
Here are a few examples to show alerts in different situations:
Conditional alerts
Conditional alerts are used to provide feedback to the user based on certain conditions or outcomes. For example, you can display different alert messages depending on the result of an API call. This helps users understand the success or failure of their actions.
fetchDataFromAPI().then(response => {
if (response.status === 200) {
showAlert('Data fetched successfully', 'success');
} else {
showAlert('Failed to fetch data', 'error');
}
});
Error handling
Error handling alerts are used to inform users when an error occurs during operations such as API requests or other processes. This helps users understand that something went wrong and provides troubleshooting guidance.
try {
// Some code that might throw an error
} catch (error) {
showAlert('An error occurred: ' + error.message, 'error');
}
Asynchronous function alerts
Asynchronous function alerts provide feedback once an asynchronous operation completes. This is useful for showing alerts after operations like navigation or data fetching have finished. For example, if you need to show an alert after a query execution is completed, use async-await to ensure the query has finished running before displaying the alert.
async function logout() {
await getUsers.run(); // Wait for the query execution to complete
showAlert('Logout Successful', 'success'); // Show the message after execution is complete
}